We will go over one of the most important data structures in Python, Lists.

Lists are ordered and start at index of zero. If you type this line at the In prompt, you can create an empty List.

You can use the type() function to find what kind of Python object, a particular variable represents. Thus L, which was created previously, is found to be indeed a List.

For Lists, one the most useful functions is to know their length. We can find the length of a List, using the len() function. We find that it is indeed 0, as our List is empty.

Here we define a List of 3 different elements.

We can extract individual elements of the List using the index number inside square brackets. In Python, this holds even for other data structures.

The individual elements within a List may be any Python object. Here we have an integer, floating-point number and a string.

To add an element to an array, the common way is using the append() function. We can also use the plus operator to join lists.

It is possible to create nested Lists, that is Lists within Lists.

To extract an element from a nested list, you will have to have two indices and two separate bracket pairs.

Negative indices can be used as well. For example -1 refers to the last element and -2 is the second to last element.

The common way to check if a value is present in a List is to use the in command. It will return either True or False.
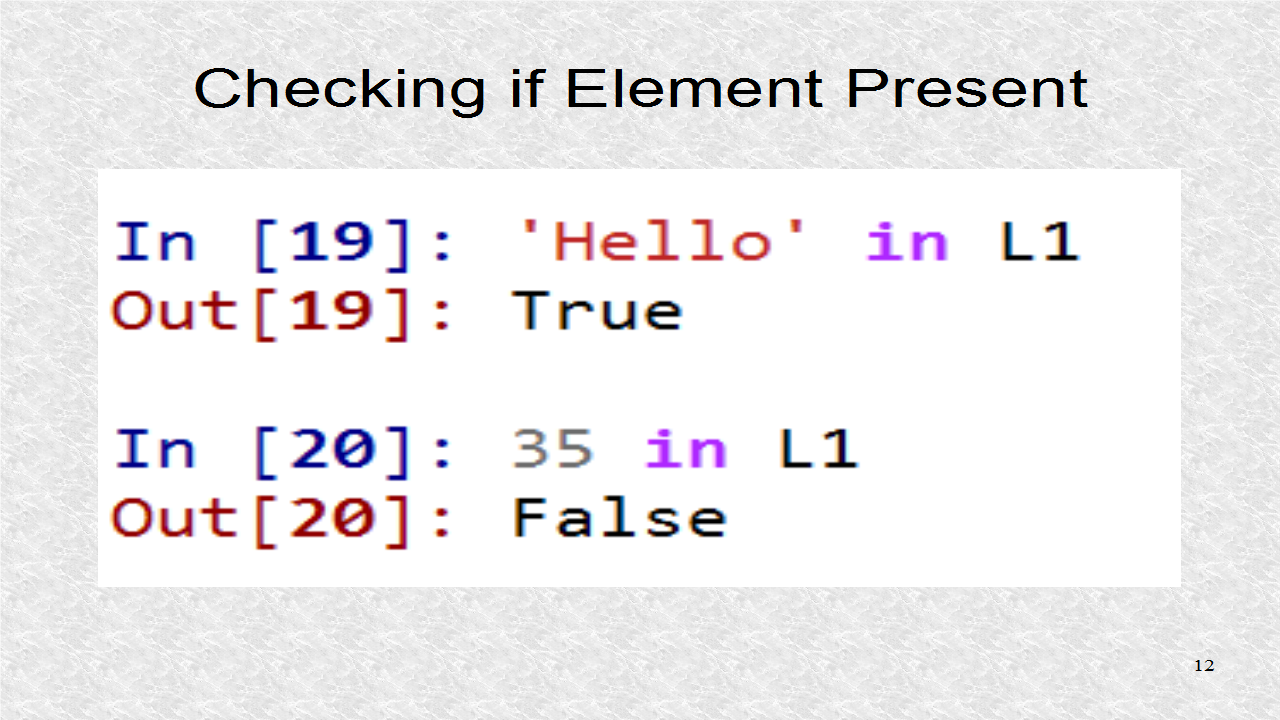
If we want to iterate over a List, we can use the for loop. Here the block that is executed for each element is just one line long, mainly a print statement.

Slicing refers to extracting a List from another List. For slicing we have to give two numbers, the first number gives the starting element of the new List and the second number gives the boundary, the first value that is not in the new List. If either of the two numbers is not listed, 0 is assumed (for first number), or List length (for second number).

We can also use negative values in slicing. This is useful if we do not know what is the size of the List.

The multiply symbol, applied to a List, refers to repeating a List.

Two of the important List functions are count() and index(). count() returns how many elements are of a particular value, while index() returns the first index found for the given value.

If count() is used with a value not in List, it returns 0, while index() will return an error. Errors are also known as Exceptions.

Strings are very similar to Lists of characters.

However individual string characters can not be changed. This is called the immutable property in Python.

If we want to really create a List from a String value, we have to use the list() function. Now individual members can be changed as a List is mutable. Finally we can use the string function, join() to create a string from a List of characters. For the join() function we have to start with quotes so it knows we want to create a String.

You may go to pythonaudio.blogspot.com to see the slides. To see a larger image of the slide, you can click on them at that page, which provides easy navigation controls. The text for the audio of the slides as well as any relevant source code is also on that page.

This is the video of Tutorial 7:
nice article for beginners.thank you.
ReplyDeletejavacodegeeks
welookups python